SinglePixelCamera
- class deepinv.physics.SinglePixelCamera(m, img_shape, fast=True, device='cpu', dtype=torch.float32, rng: Generator | None = None, **kwargs)[source]
Bases:
DecomposablePhysics
Single pixel imaging camera.
Linear imaging operator with binary entries.
If
fast=False
, the operator uses a 2D subsampled hadamard transform, which keeps the first \(m\) modes according to the sequency ordering. In this case, the images should have a size which is a power of 2.If
fast=False
, the operator is a random iid binary matrix with equal probability of \(1/\sqrt{m}\) or \(-1/\sqrt{m}\).Both options allow for an efficient singular value decomposition (see
deepinv.physics.DecomposablePhysics()
) The operator is always applied independently across channels.It is recommended to use
fast=True
for image sizes bigger than 32 x 32, since the forward computation withfast=False
has an \(O(mn)\) complexity, whereas withfast=True
it has an \(O(n \log n)\) complexity.An existing operator can be loaded from a saved
.pth
file viaself.load_state_dict(save_path)
, in a similar fashion totorch.nn.Module()
.- Parameters:
m (int) – number of single pixel measurements per acquisition (m).
img_shape (tuple) – shape (C, H, W) of images.
fast (bool) – The operator is iid binary if false, otherwise A is a 2D subsampled hadamard transform.
device (str) – Device to store the forward matrix.
rng (torch.Generator (Optional)) – a pseudorandom random number generator for the parameter generation. If
None
, the default Generator of PyTorch will be used.
- Examples:
SinglePixelCamera operators with 16 binary patterns for 32x32 image:
>>> from deepinv.physics import SinglePixelCamera >>> seed = torch.manual_seed(0) # Random seed for reproducibility >>> x = torch.randn((1, 1, 32, 32)) # Define random 32x32 image >>> physics = SinglePixelCamera(m=16, img_shape=(1, 32, 32), fast=True) >>> torch.sum(physics.mask).item() # Number of measurements 48.0 >>> torch.round(physics(x)[:, :, :3, :3]).abs() # Compute measurements tensor([[[[1., 0., 0.], [0., 0., 0.], [0., 0., 0.]]]])
Examples using SinglePixelCamera
:
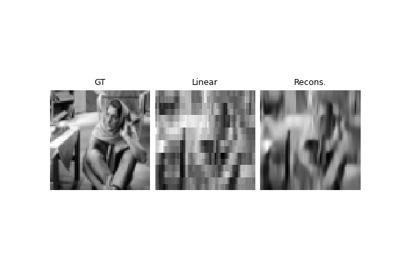
PnP with custom optimization algorithm (Condat-Vu Primal-Dual)