MRI
- class deepinv.physics.MRI(mask: Tensor | None = None, img_size: tuple | None = (320, 320), device='cpu', **kwargs)[source]
Bases:
DecomposablePhysics
Single-coil accelerated magnetic resonance imaging.
The linear operator operates in 2D slices and is defined as
\[y = SFx\]where \(S\) applies a mask (subsampling operator), and \(F\) is the 2D discrete Fourier Transform. This operator has a simple singular value decomposition, so it inherits the structure of
deepinv.physics.DecomposablePhysics()
and thus have a fast pseudo-inverse and prox operators.The complex images \(x\) and measurements \(y\) should be of size (B, 2, H, W) where the first channel corresponds to the real part and the second channel corresponds to the imaginary part.
A fixed mask can be set at initialisation, or a new mask can be set either at forward (using
physics(x, mask=mask)
) or usingupdate_parameters
.Note
We provide various random mask generators (e.g. Cartesian undersampling) that can be used directly with this physics. See e.g.
deepinv.physics.generator.mri.RandomMaskGenerator
- Parameters:
mask (torch.Tensor) – binary mask, where 1s represent sampling locations, and 0s otherwise. The mask size can either be (H,W), (C,H,W), or (B,C,H,W) where H, W are the image height and width, C is channels (typically 2) and B is batch size.
img_size (tuple) – if mask not specified, blank mask of ones is created using
img_size
, whereimg_size
can be of any shape specified above. If mask provided,img_size
is ignored.device (torch.device) – cpu or gpu.
- Examples:
Single-coil accelerated MRI operator with subsampling mask:
>>> from deepinv.physics import MRI >>> seed = torch.manual_seed(0) # Random seed for reproducibility >>> x = torch.randn(1, 2, 2, 2) # Define random 2x2 image >>> mask = 1 - torch.eye(2) # Define subsampling mask >>> physics = MRI(mask=mask) # Define mask at initialisation >>> physics(x) tensor([[[[ 0.0000, -1.4290], [ 0.4564, -0.0000]], [[ 0.0000, 1.8622], [ 0.0603, -0.0000]]]]) >>> physics = MRI(img_size=x.shape) # No subsampling >>> physics(x) tensor([[[[ 2.2908, -1.4290], [ 0.4564, -0.1814]], [[ 0.3744, 1.8622], [ 0.0603, -0.6209]]]]) >>> physics.update_parameters(mask=mask) # Update mask on the fly >>> physics(x) tensor([[[[ 0.0000, -1.4290], [ 0.4564, -0.0000]], [[ 0.0000, 1.8622], [ 0.0603, -0.0000]]]])
Examples using MRI
:
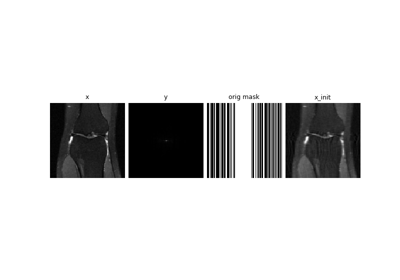
Self-supervised MRI reconstruction with Artifact2Artifact
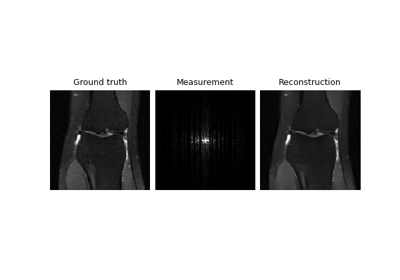
Self-supervised learning with Equivariant Imaging for MRI.