MotionBlurGenerator
- class deepinv.physics.generator.MotionBlurGenerator(psf_size: tuple, num_channels: int = 1, rng: Generator | None = None, device: str = 'cpu', dtype: type = torch.float32, l: float = 0.3, sigma: float = 0.25, n_steps: int = 1000)[source]
Bases:
PSFGenerator
Random motion blur generator.
See https://arxiv.org/pdf/1406.7444.pdf for more details.
A blur trajectory is generated by sampling both its x- and y-coordinates independently from a Gaussian Process with a Matérn 3/2 covariance function.
\[f_x(t), f_y(t) \sim \mathcal{GP}(0, k(t, t'))\]where \(k\) is defined as
\[k(t, s) = \sigma^2 \left( 1 + \frac{\sqrt{5} |t -s|}{l} + \frac{5 (t-s)^2}{3 l^2} \right) \exp \left(-\frac{\sqrt{5} |t-s|}{l}\right)\]- Parameters:
psf_size (tuple) – the shape of the generated PSF in 2D, should be (kernel_size, kernel_size)
num_channels (int) – number of images channels. Defaults to 1.
l (float) – the length scale of the trajectory, defaults to 0.3
sigma (float) – the standard deviation of the Gaussian Process, defaults to 0.25
n_steps (int) – the number of points in the trajectory, defaults to 1000
- Examples:
>>> from deepinv.physics.generator import MotionBlurGenerator >>> generator = MotionBlurGenerator((5, 5), num_channels=1) >>> blur = generator.step() # dict_keys(['filter']) >>> print(blur['filter'].shape) torch.Size([1, 1, 5, 5])
- f_matern(batch_size: int = 1, sigma: float | None = None, l: float | None = None)[source]
Generates the trajectory.
- matern_kernel(diff, sigma: float | None = None, l: float | None = None)[source]
Compute the Matérn 3/2 covariance.
- Parameters:
diff (torch.Tensor) – the difference t - s
sigma (float) – the standard deviation of the Gaussian Process
l (float) – the length scale of the trajectory
- step(batch_size: int = 1, sigma: float | None = None, l: float | None = None, seed: int | None = None)[source]
Generate a random motion blur PSF with parameters \(\sigma\) and \(l\)
- Parameters:
- Returns:
dictionary with key ‘filter’: the generated PSF of shape (batch_size, 1, psf_size[0], psf_size[1])
Examples using MotionBlurGenerator
:
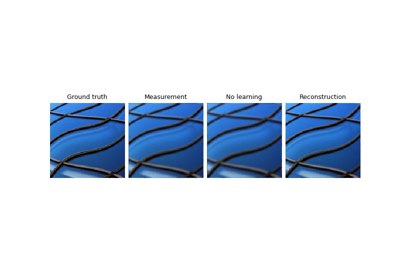
Imaging inverse problems with adversarial networks