Note
Go to the end to download the full example code.
Imaging inverse problems with adversarial networks#
This example shows you how to train various networks using adversarial
training for deblurring problems. We demonstrate running training and
inference using a conditional GAN (i.e. DeblurGAN), CSGM, AmbientGAN and
UAIR implemented in the library, and how to simply train
your own GAN by using deepinv.training.AdversarialTrainer
. These
examples can also be easily extended to train more complicated GANs such
as CycleGAN.
This example is based on the following papers:
Kupyn et al., DeblurGAN: Blind Motion Deblurring Using Conditional Adversarial Networks
Bora et al., Compressed Sensing using Generative Models (CSGM)
Bora et al., AmbientGAN: Generative models from lossy measurements
Pajot et al., Unsupervised Adversarial Image Reconstruction
Adversarial networks are characterised by the addition of an adversarial loss \(\mathcal{L}_\text{adv}\) to the standard reconstruction loss:
where \(D(\cdot)\) is the discriminator model, \(x\) is the reference image, \(\hat x\) is the estimated reconstruction, \(q(\cdot)\) is a quality function (e.g \(q(x)=x\) for WGAN). Training alternates between generator \(G\) and discriminator \(D\) in a minimax game. When there are no ground truths (i.e. unsupervised), this may be defined on the measurements \(y\) instead.
from pathlib import Path
import torch
from torch.utils.data import DataLoader, random_split
from torchvision.datasets import ImageFolder
from torchvision.transforms import Compose, ToTensor, CenterCrop, Resize
from torchvision.datasets.utils import download_and_extract_archive
import deepinv as dinv
from deepinv.loss import adversarial
from deepinv.utils.demo import get_data_home
from deepinv.physics.generator import MotionBlurGenerator
device = dinv.utils.get_freer_gpu() if torch.cuda.is_available() else "cpu"
BASE_DIR = Path(".")
DATA_DIR = BASE_DIR / "measurments"
ORGINAL_DATA_DIR = get_data_home() / "Urban100"
Generate dataset#
In this example we use the Urban100 dataset resized to 128x128. We apply random
motion blur physics using
deepinv.physics.generator.MotionBlurGenerator
, and save the data
using deepinv.datasets.generate_dataset()
.
physics = dinv.physics.Blur(padding="circular", device=device)
blur_generator = MotionBlurGenerator((11, 11))
dataset = dinv.datasets.Urban100HR(
root=ORGINAL_DATA_DIR,
download=True,
transform=Compose([ToTensor(), Resize(256), CenterCrop(128)]),
)
train_dataset, test_dataset = random_split(dataset, (0.8, 0.2))
# Generate data pairs x,y offline using a physics generator
dataset_path = dinv.datasets.generate_dataset(
train_dataset=train_dataset,
test_dataset=test_dataset,
physics=physics,
physics_generator=blur_generator,
device=device,
save_dir=DATA_DIR,
batch_size=1,
)
train_dataloader = DataLoader(
dinv.datasets.HDF5Dataset(dataset_path, train=True), shuffle=True
)
test_dataloader = DataLoader(
dinv.datasets.HDF5Dataset(dataset_path, train=False), shuffle=False
)
0%| | 0/135388067 [00:00<?, ?it/s]
4%|▍ | 5.75M/129M [00:00<00:02, 60.2MB/s]
24%|██▍ | 31.5M/129M [00:00<00:00, 183MB/s]
44%|████▍ | 57.1M/129M [00:00<00:00, 222MB/s]
65%|██████▍ | 83.4M/129M [00:00<00:00, 243MB/s]
83%|████████▎ | 107M/129M [00:00<00:00, 237MB/s]
100%|██████████| 129M/129M [00:00<00:00, 230MB/s]
Extracting: 0%| | 0/101 [00:00<?, ?it/s]
Extracting: 21%|██ | 21/101 [00:00<00:00, 203.41it/s]
Extracting: 48%|████▊ | 48/101 [00:00<00:00, 239.63it/s]
Extracting: 71%|███████▏ | 72/101 [00:00<00:00, 223.37it/s]
Extracting: 94%|█████████▍| 95/101 [00:00<00:00, 219.28it/s]
Extracting: 100%|██████████| 101/101 [00:00<00:00, 221.34it/s]
Dataset has been successfully downloaded.
Dataset has been saved at measurments/dinv_dataset0.h5
Define models#
We first define reconstruction network (i.e conditional generator) and discriminator network to use for adversarial training. For demonstration we use a simple U-Net as the reconstruction network and the discriminator from PatchGAN, but these can be replaced with any architecture e.g transformers, unrolled etc. Further discriminator models are in adversarial models.
def get_models(model=None, D=None, lr_g=1e-4, lr_d=1e-4, device=device):
if model is None:
model = dinv.models.UNet(
in_channels=3,
out_channels=3,
scales=2,
circular_padding=True,
batch_norm=False,
).to(device)
if D is None:
D = dinv.models.PatchGANDiscriminator(n_layers=2, batch_norm=False).to(device)
optimizer = dinv.training.adversarial.AdversarialOptimizer(
torch.optim.Adam(model.parameters(), lr=lr_g, weight_decay=1e-8),
torch.optim.Adam(D.parameters(), lr=lr_d, weight_decay=1e-8),
)
scheduler = dinv.training.adversarial.AdversarialScheduler(
torch.optim.lr_scheduler.StepLR(optimizer.G, step_size=5, gamma=0.9),
torch.optim.lr_scheduler.StepLR(optimizer.D, step_size=5, gamma=0.9),
)
return model, D, optimizer, scheduler
Conditional GAN training#
Conditional GANs (Kupyn et al., DeblurGAN: Blind Motion Deblurring Using Conditional Adversarial Networks) are a type of GAN where the generator is conditioned on a label or input. In the context of imaging, this can be used to generate images from a given measurement. In this example, we use a simple U-Net as the generator and a PatchGAN discriminator. The forward pass of the generator is given by:
Conditional GAN forward pass:
Conditional GAN loss:
where \(\mathcal{L}_\text{sup}\) is a supervised loss such as pixel-wise MSE or VGG Perceptual Loss.
We next define pixel-wise and adversarial losses as defined above. We use the MSE for the supervised pixel-wise metric for simplicity but this can be easily replaced with a perceptual loss if desired.
We are now ready to train the networks using deepinv.training.AdversarialTrainer
.
We load the pretrained models that were trained in the exact same way after 50 epochs,
and fine-tune the model for 1 epoch for a quick demo.
You can find the pretrained models on HuggingFace https://huggingface.co/deepinv/adversarial-demo.
To train from scratch, simply comment out the model loading code and increase the number of epochs.
ckpt = torch.hub.load_state_dict_from_url(
dinv.models.utils.get_weights_url("adversarial-demo", "deblurgan_model.pth"),
map_location=lambda s, _: s,
)
G.load_state_dict(ckpt["state_dict"])
D.load_state_dict(ckpt["state_dict_D"])
optimizer.load_state_dict(ckpt["optimizer"])
trainer = dinv.training.AdversarialTrainer(
model=G,
D=D,
physics=physics,
train_dataloader=train_dataloader,
eval_dataloader=test_dataloader,
epochs=1,
losses=loss_g,
losses_d=loss_d,
optimizer=optimizer,
scheduler=scheduler,
verbose=True,
show_progress_bar=False,
save_path=None,
device=device,
)
G = trainer.train()
Downloading: "https://huggingface.co/deepinv/adversarial-demo/resolve/main/deblurgan_model.pth?download=true" to /home/runner/.cache/torch/hub/checkpoints/deblurgan_model.pth
0%| | 0.00/12.7M [00:00<?, ?B/s]
39%|███▉ | 5.00M/12.7M [00:00<00:00, 52.3MB/s]
100%|██████████| 12.7M/12.7M [00:00<00:00, 97.0MB/s]
The model has 444867 trainable parameters
/home/runner/work/deepinv/deepinv/deepinv/training/adversarial.py:166: UserWarning: optimizer_step_multi_dataset parameter of Trainer is should be set to `False` when using adversarial trainer. Automatically setting it to `False`.
warnings.warn(
Train epoch 0: SupLoss=0.004, SupAdversarialGeneratorLoss=0.003, TotalLoss=0.007, PSNR=25.235
Eval epoch 0: PSNR=25.43
Best model saved at epoch 1
Test the trained model and plot the results. We compare to the pseudo-inverse as a baseline.
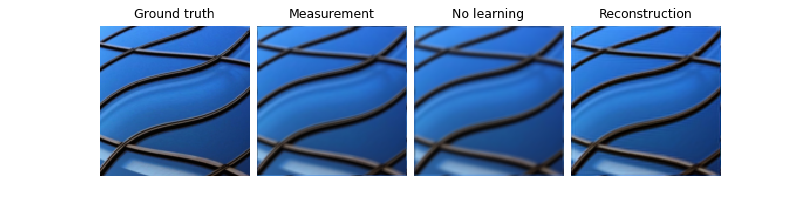
Eval epoch 0: PSNR=25.43, PSNR no learning=23.111
Test results:
PSNR no learning: 23.111 +- 2.733
PSNR: 25.430 +- 3.299
{'PSNR no learning': np.float64(23.110940551757814), 'PSNR no learning_std': np.float64(2.7325177722415908), 'PSNR': np.float64(25.430047607421876), 'PSNR_std': np.float64(3.298856479885389)}
UAIR training#
Unsupervised Adversarial Image Reconstruction (UAIR) (Pajot et al., Unsupervised Adversarial Image Reconstruction) is a method for solving inverse problems using generative models. In this example, we use a simple U-Net as the generator and discriminator, and train using the adversarial loss. The forward pass of the generator is defined as:
UAIR forward pass:
UAIR loss:
We next load the models and construct losses as defined above.
We are now ready to train the networks using deepinv.training.AdversarialTrainer
.
Like above, we load a pretrained model trained in the exact same way for 50 epochs,
and fine-tune here for a quick demo with 1 epoch.
ckpt = torch.hub.load_state_dict_from_url(
dinv.models.utils.get_weights_url("adversarial-demo", "uair_model.pth"),
map_location=lambda s, _: s,
)
G.load_state_dict(ckpt["state_dict"])
D.load_state_dict(ckpt["state_dict_D"])
optimizer.load_state_dict(ckpt["optimizer"])
trainer = dinv.training.AdversarialTrainer(
model=G,
D=D,
physics=physics,
train_dataloader=train_dataloader,
eval_dataloader=test_dataloader,
epochs=1,
losses=loss_g,
losses_d=loss_d,
optimizer=optimizer,
scheduler=scheduler,
verbose=True,
show_progress_bar=False,
save_path=None,
device=device,
)
G = trainer.train()
Downloading: "https://huggingface.co/deepinv/adversarial-demo/resolve/main/uair_model.pth?download=true" to /home/runner/.cache/torch/hub/checkpoints/uair_model.pth
0%| | 0.00/12.7M [00:00<?, ?B/s]
2%|▏ | 256k/12.7M [00:00<00:05, 2.48MB/s]
15%|█▍ | 1.88M/12.7M [00:00<00:01, 10.8MB/s]
88%|████████▊ | 11.1M/12.7M [00:00<00:00, 49.3MB/s]
100%|██████████| 12.7M/12.7M [00:00<00:00, 41.8MB/s]
The model has 444867 trainable parameters
Train epoch 0: TotalLoss=0.144, PSNR=24.253
Eval epoch 0: PSNR=23.978
Best model saved at epoch 1
Test the trained model and plot the results:
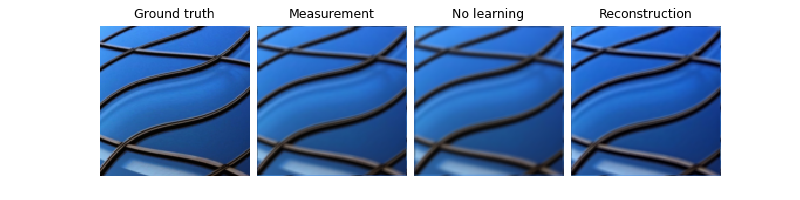
Eval epoch 0: PSNR=23.978, PSNR no learning=23.111
Test results:
PSNR no learning: 23.111 +- 2.733
PSNR: 23.978 +- 2.705
{'PSNR no learning': np.float64(23.110940551757814), 'PSNR no learning_std': np.float64(2.7325177722415908), 'PSNR': np.float64(23.978289794921874), 'PSNR_std': np.float64(2.7051143970949734)}
CSGM / AmbientGAN training#
Compressed Sensing using Generative Models (CSGM) and AmbientGAN are two methods for solving inverse problems using generative models. CSGM (Bora et al., Compressed Sensing using Generative Models) uses a generative model to solve the inverse problem by optimising the latent space of the generator. AmbientGAN (Bora et al., AmbientGAN: Generative models from lossy measurements) uses a generative model to solve the inverse problem by optimising the measurements themselves. Both methods are trained using an adversarial loss; the main difference is that CSGM requires a ground truth dataset (supervised loss), while AmbientGAN does not (unsupervised loss).
In this example, we use a DCGAN as the generator and discriminator, and train using the adversarial loss. The forward pass of the generator is given by:
CSGM forward pass at train time:
CSGM/AmbientGAN forward pass at eval time:
CSGM loss:
AmbientGAN loss (where \(\forw{\cdot}\) is the physics):
We next load the models and construct losses as defined above.
G = dinv.models.CSGMGenerator(
dinv.models.DCGANGenerator(output_size=128, nz=100, ngf=32), inf_tol=1e-2
).to(device)
D = dinv.models.DCGANDiscriminator(ndf=32).to(device)
_, _, optimizer, scheduler = get_models(
model=G, D=D, lr_g=2e-4, lr_d=2e-4
) # learning rates from original paper
# For AmbientGAN:
loss_g = adversarial.UnsupAdversarialGeneratorLoss(device=device)
loss_d = adversarial.UnsupAdversarialDiscriminatorLoss(device=device)
# For CSGM:
loss_g = adversarial.SupAdversarialGeneratorLoss(device=device)
loss_d = adversarial.SupAdversarialDiscriminatorLoss(device=device)
As before, we can now train our models. Since inference is very slow for CSGM/AmbientGAN as it requires an optimisation, we only do one evaluation at the end. Note the train PSNR is meaningless as this generative model is trained on random latents. Like above, we load a pretrained model trained in the exact same way for 50 epochs, and fine-tune here for a quick demo with 1 epoch.
ckpt = torch.hub.load_state_dict_from_url(
dinv.models.utils.get_weights_url("adversarial-demo", "csgm_model.pth"),
map_location=lambda s, _: s,
)
G.load_state_dict(ckpt["state_dict"])
D.load_state_dict(ckpt["state_dict_D"])
optimizer.load_state_dict(ckpt["optimizer"])
trainer = dinv.training.AdversarialTrainer(
model=G,
D=D,
physics=physics,
train_dataloader=train_dataloader,
epochs=1,
losses=loss_g,
losses_d=loss_d,
optimizer=optimizer,
scheduler=scheduler,
verbose=True,
show_progress_bar=False,
save_path=None,
device=device,
)
G = trainer.train()
Downloading: "https://huggingface.co/deepinv/adversarial-demo/resolve/main/csgm_model.pth?download=true" to /home/runner/.cache/torch/hub/checkpoints/csgm_model.pth
0%| | 0.00/49.3M [00:00<?, ?B/s]
1%| | 256k/49.3M [00:00<00:19, 2.58MB/s]
4%|▍ | 2.00M/49.3M [00:00<00:04, 11.7MB/s]
22%|██▏ | 10.6M/49.3M [00:00<00:00, 47.4MB/s]
42%|████▏ | 20.8M/49.3M [00:00<00:00, 69.9MB/s]
62%|██████▏ | 30.8M/49.3M [00:00<00:00, 82.3MB/s]
86%|████████▋ | 42.6M/49.3M [00:00<00:00, 96.3MB/s]
100%|██████████| 49.3M/49.3M [00:00<00:00, 78.1MB/s]
The model has 3608000 trainable parameters
Train epoch 0: TotalLoss=0.007, PSNR=9.136
Eventually, we run evaluation of the generative model by running test-time optimisation using test measurements. Note that we do not get great results as CSGM / AmbientGAN relies on large datasets of diverse samples, and we run the optimisation to a relatively high tolerance for speed. Improve the results by running the optimisation for longer.
Eval epoch 0: PSNR=10.25, PSNR no learning=23.111
Test results:
PSNR no learning: 23.111 +- 2.733
PSNR: 10.250 +- 1.120
{'PSNR no learning': np.float64(23.110940551757814), 'PSNR no learning_std': np.float64(2.7325177722415908), 'PSNR': np.float64(10.24966812133789), 'PSNR_std': np.float64(1.1202718281006854)}
Total running time of the script: (1 minutes 24.679 seconds)