Note
Go to the end to download the full example code.
Patch priors for limited-angle computed tomography#
In this example we use patch priors for limited angle computed tomography. More precisely, we consider the inverse problem \(y = \mathrm{noisy}(Ax)\), where \(A\) is the discretized Radon transform with \(100\) equispace angles between 20 and 160 degrees. For the reconstruction, we minimize the variational problem
Here, the regularizier \(g\) is explicitly defined as
where \(P_i\) is the linear operator which extracts the \(i\)-th patch from the image \(x\) and \(h\) is a regularizer on the space of patches. We consider the following two choices of \(h\):
The expected patch log-likelihood (EPLL) prior was proposed in “From learning models of natural image patches to whole image restoration”. It sets \(h(x)=-\log(p_\theta(x))\), where \(p_\theta\) is the probability density function of a Gaussian mixture model. The parameters \(\theta\) are estimated a-priori on a (possibly small) data set of training patches using an expectation maximization algorithm. In contrast to the original paper by Zoran and Weiss, we minimize the arising variational problem by simply applying the Adam optimizers. For an example for using the (approximated) half-quadratic splitting algorithm proposed by Zoran and Weiss, we refer to the denoising example…
The patch normalizing flow regularizer (PatchNR) was proposed in “PatchNR: learning from very few images by patch normalizing flow regularization”. It models \(h(x)=-\log(p_{\theta}(x))\) as negative log-likelihood function of a probaility density function \(p_\theta={\mathcal{T}_\theta}_\#\mathcal{N}(0,I)\) which is given as the push-forward measure of a standard normal distribution under a normalizing flow (invertible neural network) \(\mathcal{T}_\theta\).
import torch
from torch.utils.data import DataLoader
from deepinv.datasets import PatchDataset
from deepinv import Trainer
from deepinv.physics import LogPoissonNoise, Tomography, Denoising, UniformNoise
from deepinv.optim import LogPoissonLikelihood, PatchPrior, PatchNR, EPLL
from deepinv.loss.metric import PSNR
from deepinv.utils import plot
from deepinv.utils.demo import load_torch_url
from tqdm import tqdm
device = "cuda" if torch.cuda.is_available() else "cpu"
dtype = torch.float32
Load training and test images#
Here, we use downsampled images from the “LoDoPaB-CT dataset”. Moreover, we define the size of the used patches and generate the dataset of patches in the training images.
url = "https://huggingface.co/datasets/deepinv/LoDoPaB-CT_toy/resolve/main/LoDoPaB-CT_small.pt"
dataset = load_torch_url(url)
train_imgs = dataset["train_imgs"].to(device)
test_imgs = dataset["test_imgs"].to(device)
img_size = train_imgs.shape[-1]
patch_size = 3
verbose = True
train_dataset = PatchDataset(train_imgs, patch_size=patch_size, transforms=None)
Set parameters for EPLL and PatchNR#
For PatchNR, we choose the number of hidden neurons in the subnetworks and for the training batch size and number of epochs. For EPLL, we set the number of mixture components and the maximum number of steps and batch size for fitting the EM algorithm.
patchnr_subnetsize = 128
patchnr_epochs = 5
patchnr_batch_size = 32
patchnr_learning_rate = 1e-4
epll_num_components = 20
epll_max_iter = 20
epll_batch_size = 10000
Training / EM algorithm#
If the parameter retrain is False, we just load pretrained weights. Set the parameter to True for retraining. On the cpu, this takes up to a couple of minutes. After training, we define the corresponding patch priors
Note
The normalizing flow training minimizes the forward Kullback-Leibler (maximum likelihood) loss function given by
\[\mathcal{L}(\theta)=\mathrm{KL}(P_X,{\mathcal{T}_\theta}_\#P_Z)= \mathbb{E}_{x\sim P_X}[p_{{\mathcal{T}_\theta}_\#P_Z}(x)]+\mathrm{const},\]where \(\mathcal{T}_\theta\) is the normalizing flow with parameters \(\theta\), latent distribution \(P_Z\), data distribution \(P_X\) and push-forward measure \({\mathcal{T}_\theta}_\#P_Z\).
retrain = False
if retrain:
model_patchnr = PatchNR(
pretrained=None,
sub_net_size=patchnr_subnetsize,
device=device,
patch_size=patch_size,
)
patchnr_dataloader = DataLoader(
train_dataset,
batch_size=patchnr_batch_size,
shuffle=True,
drop_last=True,
)
class NFTrainer(Trainer):
def compute_loss(self, physics, x, y, train=True):
logs = {}
self.optimizer.zero_grad() # Zero the gradients
# Evaluate reconstruction network
invs, jac_inv = self.model(y)
# Compute the Kullback Leibler loss
loss_total = torch.mean(
0.5 * torch.sum(invs.view(invs.shape[0], -1) ** 2, -1)
- jac_inv.view(invs.shape[0])
)
current_log = (
self.logs_total_loss_train if train else self.logs_total_loss_eval
)
current_log.update(loss_total.item())
logs[f"TotalLoss"] = current_log.avg
if train:
loss_total.backward() # Backward the total loss
self.optimizer.step() # Optimizer step
return invs, logs
optimizer = torch.optim.Adam(
model_patchnr.normalizing_flow.parameters(), lr=patchnr_learning_rate
)
trainer = NFTrainer(
model=model_patchnr.normalizing_flow,
physics=Denoising(UniformNoise(1.0 / 255.0)),
optimizer=optimizer,
train_dataloader=patchnr_dataloader,
device=device,
losses=[],
epochs=patchnr_epochs,
online_measurements=True,
verbose=verbose,
)
trainer.train()
model_epll = EPLL(
pretrained=None,
n_components=epll_num_components,
patch_size=patch_size,
device=device,
)
epll_dataloader = DataLoader(
train_dataset,
batch_size=epll_batch_size,
shuffle=True,
drop_last=False,
)
model_epll.GMM.fit(epll_dataloader, verbose=verbose, max_iters=epll_max_iter)
else:
model_patchnr = PatchNR(
pretrained="PatchNR_lodopab_small2",
sub_net_size=patchnr_subnetsize,
device=device,
patch_size=patch_size,
)
model_epll = EPLL(
pretrained="GMM_lodopab_small2",
n_components=epll_num_components,
patch_size=patch_size,
device=device,
)
patchnr_prior = PatchPrior(model_patchnr, patch_size=patch_size)
epll_prior = PatchPrior(model_epll.negative_log_likelihood, patch_size=patch_size)
Downloading: "https://drive.google.com/uc?export=download&id=1Z2us9ZHjDGOlU6r1Jee0s2BBej2XV5-i" to /home/runner/.cache/torch/hub/checkpoints/PatchNR_lodopab_small.pt
0%| | 0.00/739k [00:00<?, ?B/s]
100%|██████████| 739k/739k [00:00<00:00, 11.7MB/s]
Downloading: "https://huggingface.co/deepinv/EPLL/resolve/main/GMM_lodopab_small2.pt?download=true" to /home/runner/.cache/torch/hub/checkpoints/GMM_lodopab_small2.pt
0%| | 0.00/28.9k [00:00<?, ?B/s]
100%|██████████| 28.9k/28.9k [00:00<00:00, 13.2MB/s]
Definition of forward operator and noise model#
The training depends only on the image domain or prior distribution. For the reconstruction, we now define forward operator and noise model. For the noise model, we use log-Poisson noise as suggested for the LoDoPaB dataset. Then, we generate an observation by applying the physics and compute the filtered backprojection.
mu = 1 / 50.0 * (362.0 / img_size)
N0 = 1024.0
num_angles = 100
noise_model = LogPoissonNoise(mu=mu, N0=N0)
data_fidelity = LogPoissonLikelihood(mu=mu, N0=N0)
angles = torch.linspace(20, 160, steps=num_angles)
physics = Tomography(
img_width=img_size, angles=angles, device=device, noise_model=noise_model
)
observation = physics(test_imgs)
fbp = physics.A_dagger(observation)
Reconstruction loop#
We define a reconstruction loop for minimizing the variational problem using the Adam optimizer. As initialization, we choose the filtered backprojection.
optim_steps = 200
lr_variational_problem = 0.02
def minimize_variational_problem(prior, lam):
imgs = fbp.detach().clone()
imgs.requires_grad_(True)
optimizer = torch.optim.Adam([imgs], lr=lr_variational_problem)
for i in (progress_bar := tqdm(range(optim_steps))):
optimizer.zero_grad()
loss = data_fidelity(imgs, observation, physics).mean() + lam * prior(imgs)
loss.backward()
optimizer.step()
progress_bar.set_description("Step {}".format(i + 1))
return imgs.detach()
Run and plot#
Finally, we run the reconstruction loop for both priors and plot the results. The regularization parameter is roughly choosen by a grid search but not fine-tuned
lam_patchnr = 120.0
lam_epll = 120.0
recon_patchnr = minimize_variational_problem(patchnr_prior, lam_patchnr)
recon_epll = minimize_variational_problem(epll_prior, lam_epll)
psnr_fbp = PSNR()(fbp, test_imgs).item()
psnr_patchnr = PSNR()(recon_patchnr, test_imgs).item()
psnr_epll = PSNR()(recon_epll, test_imgs).item()
print("PSNRs:")
print("Filtered Backprojection: {0:.2f}".format(psnr_fbp))
print("EPLL: {0:.2f}".format(psnr_epll))
print("PatchNR: {0:.2f}".format(psnr_patchnr))
plot(
[
test_imgs,
fbp.clip(0, 1),
recon_epll.clip(0, 1),
recon_patchnr.clip(0, 1),
],
["Ground truth", "Filtered Backprojection", "EPLL", "PatchNR"],
)
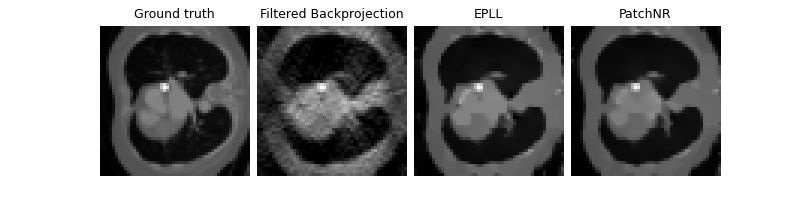
0%| | 0/200 [00:00<?, ?it/s]
Step 1: 0%| | 0/200 [00:00<?, ?it/s]
Step 2: 0%| | 0/200 [00:00<?, ?it/s]
Step 3: 0%| | 0/200 [00:00<?, ?it/s]
Step 3: 2%|▏ | 3/200 [00:00<00:09, 20.83it/s]
Step 4: 2%|▏ | 3/200 [00:00<00:09, 20.83it/s]
Step 5: 2%|▏ | 3/200 [00:00<00:09, 20.83it/s]
Step 6: 2%|▏ | 3/200 [00:00<00:09, 20.83it/s]
Step 6: 3%|▎ | 6/200 [00:00<00:09, 20.89it/s]
Step 7: 3%|▎ | 6/200 [00:00<00:09, 20.89it/s]
Step 8: 3%|▎ | 6/200 [00:00<00:09, 20.89it/s]
Step 9: 3%|▎ | 6/200 [00:00<00:09, 20.89it/s]
Step 9: 4%|▍ | 9/200 [00:00<00:08, 21.53it/s]
Step 10: 4%|▍ | 9/200 [00:00<00:08, 21.53it/s]
Step 11: 4%|▍ | 9/200 [00:00<00:08, 21.53it/s]
Step 12: 4%|▍ | 9/200 [00:00<00:08, 21.53it/s]
Step 12: 6%|▌ | 12/200 [00:00<00:08, 21.84it/s]
Step 13: 6%|▌ | 12/200 [00:00<00:08, 21.84it/s]
Step 14: 6%|▌ | 12/200 [00:00<00:08, 21.84it/s]
Step 15: 6%|▌ | 12/200 [00:00<00:08, 21.84it/s]
Step 15: 8%|▊ | 15/200 [00:00<00:08, 22.09it/s]
Step 16: 8%|▊ | 15/200 [00:00<00:08, 22.09it/s]
Step 17: 8%|▊ | 15/200 [00:00<00:08, 22.09it/s]
Step 18: 8%|▊ | 15/200 [00:00<00:08, 22.09it/s]
Step 18: 9%|▉ | 18/200 [00:00<00:08, 22.20it/s]
Step 19: 9%|▉ | 18/200 [00:00<00:08, 22.20it/s]
Step 20: 9%|▉ | 18/200 [00:00<00:08, 22.20it/s]
Step 21: 9%|▉ | 18/200 [00:00<00:08, 22.20it/s]
Step 21: 10%|█ | 21/200 [00:00<00:08, 22.30it/s]
Step 22: 10%|█ | 21/200 [00:01<00:08, 22.30it/s]
Step 23: 10%|█ | 21/200 [00:01<00:08, 22.30it/s]
Step 24: 10%|█ | 21/200 [00:01<00:08, 22.30it/s]
Step 24: 12%|█▏ | 24/200 [00:01<00:07, 22.09it/s]
Step 25: 12%|█▏ | 24/200 [00:01<00:07, 22.09it/s]
Step 26: 12%|█▏ | 24/200 [00:01<00:07, 22.09it/s]
Step 27: 12%|█▏ | 24/200 [00:01<00:07, 22.09it/s]
Step 27: 14%|█▎ | 27/200 [00:01<00:07, 22.23it/s]
Step 28: 14%|█▎ | 27/200 [00:01<00:07, 22.23it/s]
Step 29: 14%|█▎ | 27/200 [00:01<00:07, 22.23it/s]
Step 30: 14%|█▎ | 27/200 [00:01<00:07, 22.23it/s]
Step 30: 15%|█▌ | 30/200 [00:01<00:07, 22.19it/s]
Step 31: 15%|█▌ | 30/200 [00:01<00:07, 22.19it/s]
Step 32: 15%|█▌ | 30/200 [00:01<00:07, 22.19it/s]
Step 33: 15%|█▌ | 30/200 [00:01<00:07, 22.19it/s]
Step 33: 16%|█▋ | 33/200 [00:01<00:07, 22.16it/s]
Step 34: 16%|█▋ | 33/200 [00:01<00:07, 22.16it/s]
Step 35: 16%|█▋ | 33/200 [00:01<00:07, 22.16it/s]
Step 36: 16%|█▋ | 33/200 [00:01<00:07, 22.16it/s]
Step 36: 18%|█▊ | 36/200 [00:01<00:07, 22.16it/s]
Step 37: 18%|█▊ | 36/200 [00:01<00:07, 22.16it/s]
Step 38: 18%|█▊ | 36/200 [00:01<00:07, 22.16it/s]
Step 39: 18%|█▊ | 36/200 [00:01<00:07, 22.16it/s]
Step 39: 20%|█▉ | 39/200 [00:01<00:07, 22.32it/s]
Step 40: 20%|█▉ | 39/200 [00:01<00:07, 22.32it/s]
Step 41: 20%|█▉ | 39/200 [00:01<00:07, 22.32it/s]
Step 42: 20%|█▉ | 39/200 [00:01<00:07, 22.32it/s]
Step 42: 21%|██ | 42/200 [00:01<00:07, 22.39it/s]
Step 43: 21%|██ | 42/200 [00:01<00:07, 22.39it/s]
Step 44: 21%|██ | 42/200 [00:01<00:07, 22.39it/s]
Step 45: 21%|██ | 42/200 [00:02<00:07, 22.39it/s]
Step 45: 22%|██▎ | 45/200 [00:02<00:06, 22.38it/s]
Step 46: 22%|██▎ | 45/200 [00:02<00:06, 22.38it/s]
Step 47: 22%|██▎ | 45/200 [00:02<00:06, 22.38it/s]
Step 48: 22%|██▎ | 45/200 [00:02<00:06, 22.38it/s]
Step 48: 24%|██▍ | 48/200 [00:02<00:06, 22.30it/s]
Step 49: 24%|██▍ | 48/200 [00:02<00:06, 22.30it/s]
Step 50: 24%|██▍ | 48/200 [00:02<00:06, 22.30it/s]
Step 51: 24%|██▍ | 48/200 [00:02<00:06, 22.30it/s]
Step 51: 26%|██▌ | 51/200 [00:02<00:06, 22.31it/s]
Step 52: 26%|██▌ | 51/200 [00:02<00:06, 22.31it/s]
Step 53: 26%|██▌ | 51/200 [00:02<00:06, 22.31it/s]
Step 54: 26%|██▌ | 51/200 [00:02<00:06, 22.31it/s]
Step 54: 27%|██▋ | 54/200 [00:02<00:06, 22.01it/s]
Step 55: 27%|██▋ | 54/200 [00:02<00:06, 22.01it/s]
Step 56: 27%|██▋ | 54/200 [00:02<00:06, 22.01it/s]
Step 57: 27%|██▋ | 54/200 [00:02<00:06, 22.01it/s]
Step 57: 28%|██▊ | 57/200 [00:02<00:06, 22.06it/s]
Step 58: 28%|██▊ | 57/200 [00:02<00:06, 22.06it/s]
Step 59: 28%|██▊ | 57/200 [00:02<00:06, 22.06it/s]
Step 60: 28%|██▊ | 57/200 [00:02<00:06, 22.06it/s]
Step 60: 30%|███ | 60/200 [00:02<00:06, 22.18it/s]
Step 61: 30%|███ | 60/200 [00:02<00:06, 22.18it/s]
Step 62: 30%|███ | 60/200 [00:02<00:06, 22.18it/s]
Step 63: 30%|███ | 60/200 [00:02<00:06, 22.18it/s]
Step 63: 32%|███▏ | 63/200 [00:02<00:06, 22.19it/s]
Step 64: 32%|███▏ | 63/200 [00:02<00:06, 22.19it/s]
Step 65: 32%|███▏ | 63/200 [00:02<00:06, 22.19it/s]
Step 66: 32%|███▏ | 63/200 [00:02<00:06, 22.19it/s]
Step 66: 33%|███▎ | 66/200 [00:02<00:06, 22.16it/s]
Step 67: 33%|███▎ | 66/200 [00:03<00:06, 22.16it/s]
Step 68: 33%|███▎ | 66/200 [00:03<00:06, 22.16it/s]
Step 69: 33%|███▎ | 66/200 [00:03<00:06, 22.16it/s]
Step 69: 34%|███▍ | 69/200 [00:03<00:05, 22.11it/s]
Step 70: 34%|███▍ | 69/200 [00:03<00:05, 22.11it/s]
Step 71: 34%|███▍ | 69/200 [00:03<00:05, 22.11it/s]
Step 72: 34%|███▍ | 69/200 [00:03<00:05, 22.11it/s]
Step 72: 36%|███▌ | 72/200 [00:03<00:05, 22.25it/s]
Step 73: 36%|███▌ | 72/200 [00:03<00:05, 22.25it/s]
Step 74: 36%|███▌ | 72/200 [00:03<00:05, 22.25it/s]
Step 75: 36%|███▌ | 72/200 [00:03<00:05, 22.25it/s]
Step 75: 38%|███▊ | 75/200 [00:03<00:05, 22.16it/s]
Step 76: 38%|███▊ | 75/200 [00:03<00:05, 22.16it/s]
Step 77: 38%|███▊ | 75/200 [00:03<00:05, 22.16it/s]
Step 78: 38%|███▊ | 75/200 [00:03<00:05, 22.16it/s]
Step 78: 39%|███▉ | 78/200 [00:03<00:05, 22.25it/s]
Step 79: 39%|███▉ | 78/200 [00:03<00:05, 22.25it/s]
Step 80: 39%|███▉ | 78/200 [00:03<00:05, 22.25it/s]
Step 81: 39%|███▉ | 78/200 [00:03<00:05, 22.25it/s]
Step 81: 40%|████ | 81/200 [00:03<00:05, 22.40it/s]
Step 82: 40%|████ | 81/200 [00:03<00:05, 22.40it/s]
Step 83: 40%|████ | 81/200 [00:03<00:05, 22.40it/s]
Step 84: 40%|████ | 81/200 [00:03<00:05, 22.40it/s]
Step 84: 42%|████▏ | 84/200 [00:03<00:05, 22.57it/s]
Step 85: 42%|████▏ | 84/200 [00:03<00:05, 22.57it/s]
Step 86: 42%|████▏ | 84/200 [00:03<00:05, 22.57it/s]
Step 87: 42%|████▏ | 84/200 [00:03<00:05, 22.57it/s]
Step 87: 44%|████▎ | 87/200 [00:03<00:04, 22.73it/s]
Step 88: 44%|████▎ | 87/200 [00:03<00:04, 22.73it/s]
Step 89: 44%|████▎ | 87/200 [00:04<00:04, 22.73it/s]
Step 90: 44%|████▎ | 87/200 [00:04<00:04, 22.73it/s]
Step 90: 45%|████▌ | 90/200 [00:04<00:04, 22.59it/s]
Step 91: 45%|████▌ | 90/200 [00:04<00:04, 22.59it/s]
Step 92: 45%|████▌ | 90/200 [00:04<00:04, 22.59it/s]
Step 93: 45%|████▌ | 90/200 [00:04<00:04, 22.59it/s]
Step 93: 46%|████▋ | 93/200 [00:04<00:04, 22.65it/s]
Step 94: 46%|████▋ | 93/200 [00:04<00:04, 22.65it/s]
Step 95: 46%|████▋ | 93/200 [00:04<00:04, 22.65it/s]
Step 96: 46%|████▋ | 93/200 [00:04<00:04, 22.65it/s]
Step 96: 48%|████▊ | 96/200 [00:04<00:04, 22.62it/s]
Step 97: 48%|████▊ | 96/200 [00:04<00:04, 22.62it/s]
Step 98: 48%|████▊ | 96/200 [00:04<00:04, 22.62it/s]
Step 99: 48%|████▊ | 96/200 [00:04<00:04, 22.62it/s]
Step 99: 50%|████▉ | 99/200 [00:04<00:04, 22.42it/s]
Step 100: 50%|████▉ | 99/200 [00:04<00:04, 22.42it/s]
Step 101: 50%|████▉ | 99/200 [00:04<00:04, 22.42it/s]
Step 102: 50%|████▉ | 99/200 [00:04<00:04, 22.42it/s]
Step 102: 51%|█████ | 102/200 [00:04<00:04, 22.54it/s]
Step 103: 51%|█████ | 102/200 [00:04<00:04, 22.54it/s]
Step 104: 51%|█████ | 102/200 [00:04<00:04, 22.54it/s]
Step 105: 51%|█████ | 102/200 [00:04<00:04, 22.54it/s]
Step 105: 52%|█████▎ | 105/200 [00:04<00:04, 22.62it/s]
Step 106: 52%|█████▎ | 105/200 [00:04<00:04, 22.62it/s]
Step 107: 52%|█████▎ | 105/200 [00:04<00:04, 22.62it/s]
Step 108: 52%|█████▎ | 105/200 [00:04<00:04, 22.62it/s]
Step 108: 54%|█████▍ | 108/200 [00:04<00:04, 22.68it/s]
Step 109: 54%|█████▍ | 108/200 [00:04<00:04, 22.68it/s]
Step 110: 54%|█████▍ | 108/200 [00:04<00:04, 22.68it/s]
Step 111: 54%|█████▍ | 108/200 [00:04<00:04, 22.68it/s]
Step 111: 56%|█████▌ | 111/200 [00:04<00:03, 22.76it/s]
Step 112: 56%|█████▌ | 111/200 [00:05<00:03, 22.76it/s]
Step 113: 56%|█████▌ | 111/200 [00:05<00:03, 22.76it/s]
Step 114: 56%|█████▌ | 111/200 [00:05<00:03, 22.76it/s]
Step 114: 57%|█████▋ | 114/200 [00:05<00:03, 22.76it/s]
Step 115: 57%|█████▋ | 114/200 [00:05<00:03, 22.76it/s]
Step 116: 57%|█████▋ | 114/200 [00:05<00:03, 22.76it/s]
Step 117: 57%|█████▋ | 114/200 [00:05<00:03, 22.76it/s]
Step 117: 58%|█████▊ | 117/200 [00:05<00:03, 22.77it/s]
Step 118: 58%|█████▊ | 117/200 [00:05<00:03, 22.77it/s]
Step 119: 58%|█████▊ | 117/200 [00:05<00:03, 22.77it/s]
Step 120: 58%|█████▊ | 117/200 [00:05<00:03, 22.77it/s]
Step 120: 60%|██████ | 120/200 [00:05<00:03, 22.76it/s]
Step 121: 60%|██████ | 120/200 [00:05<00:03, 22.76it/s]
Step 122: 60%|██████ | 120/200 [00:05<00:03, 22.76it/s]
Step 123: 60%|██████ | 120/200 [00:05<00:03, 22.76it/s]
Step 123: 62%|██████▏ | 123/200 [00:05<00:03, 22.30it/s]
Step 124: 62%|██████▏ | 123/200 [00:05<00:03, 22.30it/s]
Step 125: 62%|██████▏ | 123/200 [00:05<00:03, 22.30it/s]
Step 126: 62%|██████▏ | 123/200 [00:05<00:03, 22.30it/s]
Step 126: 63%|██████▎ | 126/200 [00:05<00:03, 22.45it/s]
Step 127: 63%|██████▎ | 126/200 [00:05<00:03, 22.45it/s]
Step 128: 63%|██████▎ | 126/200 [00:05<00:03, 22.45it/s]
Step 129: 63%|██████▎ | 126/200 [00:05<00:03, 22.45it/s]
Step 129: 64%|██████▍ | 129/200 [00:05<00:03, 22.43it/s]
Step 130: 64%|██████▍ | 129/200 [00:05<00:03, 22.43it/s]
Step 131: 64%|██████▍ | 129/200 [00:05<00:03, 22.43it/s]
Step 132: 64%|██████▍ | 129/200 [00:05<00:03, 22.43it/s]
Step 132: 66%|██████▌ | 132/200 [00:05<00:03, 22.36it/s]
Step 133: 66%|██████▌ | 132/200 [00:05<00:03, 22.36it/s]
Step 134: 66%|██████▌ | 132/200 [00:06<00:03, 22.36it/s]
Step 135: 66%|██████▌ | 132/200 [00:06<00:03, 22.36it/s]
Step 135: 68%|██████▊ | 135/200 [00:06<00:02, 21.72it/s]
Step 136: 68%|██████▊ | 135/200 [00:06<00:02, 21.72it/s]
Step 137: 68%|██████▊ | 135/200 [00:06<00:02, 21.72it/s]
Step 138: 68%|██████▊ | 135/200 [00:06<00:02, 21.72it/s]
Step 138: 69%|██████▉ | 138/200 [00:06<00:02, 21.97it/s]
Step 139: 69%|██████▉ | 138/200 [00:06<00:02, 21.97it/s]
Step 140: 69%|██████▉ | 138/200 [00:06<00:02, 21.97it/s]
Step 141: 69%|██████▉ | 138/200 [00:06<00:02, 21.97it/s]
Step 141: 70%|███████ | 141/200 [00:06<00:02, 22.25it/s]
Step 142: 70%|███████ | 141/200 [00:06<00:02, 22.25it/s]
Step 143: 70%|███████ | 141/200 [00:06<00:02, 22.25it/s]
Step 144: 70%|███████ | 141/200 [00:06<00:02, 22.25it/s]
Step 144: 72%|███████▏ | 144/200 [00:06<00:02, 22.36it/s]
Step 145: 72%|███████▏ | 144/200 [00:06<00:02, 22.36it/s]
Step 146: 72%|███████▏ | 144/200 [00:06<00:02, 22.36it/s]
Step 147: 72%|███████▏ | 144/200 [00:06<00:02, 22.36it/s]
Step 147: 74%|███████▎ | 147/200 [00:06<00:02, 22.37it/s]
Step 148: 74%|███████▎ | 147/200 [00:06<00:02, 22.37it/s]
Step 149: 74%|███████▎ | 147/200 [00:06<00:02, 22.37it/s]
Step 150: 74%|███████▎ | 147/200 [00:06<00:02, 22.37it/s]
Step 150: 75%|███████▌ | 150/200 [00:06<00:02, 22.57it/s]
Step 151: 75%|███████▌ | 150/200 [00:06<00:02, 22.57it/s]
Step 152: 75%|███████▌ | 150/200 [00:06<00:02, 22.57it/s]
Step 153: 75%|███████▌ | 150/200 [00:06<00:02, 22.57it/s]
Step 153: 76%|███████▋ | 153/200 [00:06<00:02, 22.64it/s]
Step 154: 76%|███████▋ | 153/200 [00:06<00:02, 22.64it/s]
Step 155: 76%|███████▋ | 153/200 [00:06<00:02, 22.64it/s]
Step 156: 76%|███████▋ | 153/200 [00:06<00:02, 22.64it/s]
Step 156: 78%|███████▊ | 156/200 [00:06<00:01, 22.64it/s]
Step 157: 78%|███████▊ | 156/200 [00:07<00:01, 22.64it/s]
Step 158: 78%|███████▊ | 156/200 [00:07<00:01, 22.64it/s]
Step 159: 78%|███████▊ | 156/200 [00:07<00:01, 22.64it/s]
Step 159: 80%|███████▉ | 159/200 [00:07<00:01, 22.67it/s]
Step 160: 80%|███████▉ | 159/200 [00:07<00:01, 22.67it/s]
Step 161: 80%|███████▉ | 159/200 [00:07<00:01, 22.67it/s]
Step 162: 80%|███████▉ | 159/200 [00:07<00:01, 22.67it/s]
Step 162: 81%|████████ | 162/200 [00:07<00:01, 22.72it/s]
Step 163: 81%|████████ | 162/200 [00:07<00:01, 22.72it/s]
Step 164: 81%|████████ | 162/200 [00:07<00:01, 22.72it/s]
Step 165: 81%|████████ | 162/200 [00:07<00:01, 22.72it/s]
Step 165: 82%|████████▎ | 165/200 [00:07<00:01, 22.76it/s]
Step 166: 82%|████████▎ | 165/200 [00:07<00:01, 22.76it/s]
Step 167: 82%|████████▎ | 165/200 [00:07<00:01, 22.76it/s]
Step 168: 82%|████████▎ | 165/200 [00:07<00:01, 22.76it/s]
Step 168: 84%|████████▍ | 168/200 [00:07<00:01, 22.34it/s]
Step 169: 84%|████████▍ | 168/200 [00:07<00:01, 22.34it/s]
Step 170: 84%|████████▍ | 168/200 [00:07<00:01, 22.34it/s]
Step 171: 84%|████████▍ | 168/200 [00:07<00:01, 22.34it/s]
Step 171: 86%|████████▌ | 171/200 [00:07<00:01, 22.36it/s]
Step 172: 86%|████████▌ | 171/200 [00:07<00:01, 22.36it/s]
Step 173: 86%|████████▌ | 171/200 [00:07<00:01, 22.36it/s]
Step 174: 86%|████████▌ | 171/200 [00:07<00:01, 22.36it/s]
Step 174: 87%|████████▋ | 174/200 [00:07<00:01, 22.44it/s]
Step 175: 87%|████████▋ | 174/200 [00:07<00:01, 22.44it/s]
Step 176: 87%|████████▋ | 174/200 [00:07<00:01, 22.44it/s]
Step 177: 87%|████████▋ | 174/200 [00:07<00:01, 22.44it/s]
Step 177: 88%|████████▊ | 177/200 [00:07<00:01, 22.59it/s]
Step 178: 88%|████████▊ | 177/200 [00:07<00:01, 22.59it/s]
Step 179: 88%|████████▊ | 177/200 [00:08<00:01, 22.59it/s]
Step 180: 88%|████████▊ | 177/200 [00:08<00:01, 22.59it/s]
Step 180: 90%|█████████ | 180/200 [00:08<00:00, 22.58it/s]
Step 181: 90%|█████████ | 180/200 [00:08<00:00, 22.58it/s]
Step 182: 90%|█████████ | 180/200 [00:08<00:00, 22.58it/s]
Step 183: 90%|█████████ | 180/200 [00:08<00:00, 22.58it/s]
Step 183: 92%|█████████▏| 183/200 [00:08<00:00, 22.51it/s]
Step 184: 92%|█████████▏| 183/200 [00:08<00:00, 22.51it/s]
Step 185: 92%|█████████▏| 183/200 [00:08<00:00, 22.51it/s]
Step 186: 92%|█████████▏| 183/200 [00:08<00:00, 22.51it/s]
Step 186: 93%|█████████▎| 186/200 [00:08<00:00, 22.50it/s]
Step 187: 93%|█████████▎| 186/200 [00:08<00:00, 22.50it/s]
Step 188: 93%|█████████▎| 186/200 [00:08<00:00, 22.50it/s]
Step 189: 93%|█████████▎| 186/200 [00:08<00:00, 22.50it/s]
Step 189: 94%|█████████▍| 189/200 [00:08<00:00, 22.44it/s]
Step 190: 94%|█████████▍| 189/200 [00:08<00:00, 22.44it/s]
Step 191: 94%|█████████▍| 189/200 [00:08<00:00, 22.44it/s]
Step 192: 94%|█████████▍| 189/200 [00:08<00:00, 22.44it/s]
Step 192: 96%|█████████▌| 192/200 [00:08<00:00, 22.26it/s]
Step 193: 96%|█████████▌| 192/200 [00:08<00:00, 22.26it/s]
Step 194: 96%|█████████▌| 192/200 [00:08<00:00, 22.26it/s]
Step 195: 96%|█████████▌| 192/200 [00:08<00:00, 22.26it/s]
Step 195: 98%|█████████▊| 195/200 [00:08<00:00, 22.23it/s]
Step 196: 98%|█████████▊| 195/200 [00:08<00:00, 22.23it/s]
Step 197: 98%|█████████▊| 195/200 [00:08<00:00, 22.23it/s]
Step 198: 98%|█████████▊| 195/200 [00:08<00:00, 22.23it/s]
Step 198: 99%|█████████▉| 198/200 [00:08<00:00, 22.24it/s]
Step 199: 99%|█████████▉| 198/200 [00:08<00:00, 22.24it/s]
Step 200: 99%|█████████▉| 198/200 [00:08<00:00, 22.24it/s]
Step 200: 100%|██████████| 200/200 [00:08<00:00, 22.35it/s]
0%| | 0/200 [00:00<?, ?it/s]
Step 1: 0%| | 0/200 [00:00<?, ?it/s]
Step 2: 0%| | 0/200 [00:00<?, ?it/s]
Step 3: 0%| | 0/200 [00:00<?, ?it/s]
Step 4: 0%| | 0/200 [00:00<?, ?it/s]
Step 5: 0%| | 0/200 [00:00<?, ?it/s]
Step 6: 0%| | 0/200 [00:00<?, ?it/s]
Step 7: 0%| | 0/200 [00:00<?, ?it/s]
Step 8: 0%| | 0/200 [00:00<?, ?it/s]
Step 9: 0%| | 0/200 [00:00<?, ?it/s]
Step 9: 4%|▍ | 9/200 [00:00<00:02, 85.09it/s]
Step 10: 4%|▍ | 9/200 [00:00<00:02, 85.09it/s]
Step 11: 4%|▍ | 9/200 [00:00<00:02, 85.09it/s]
Step 12: 4%|▍ | 9/200 [00:00<00:02, 85.09it/s]
Step 13: 4%|▍ | 9/200 [00:00<00:02, 85.09it/s]
Step 14: 4%|▍ | 9/200 [00:00<00:02, 85.09it/s]
Step 15: 4%|▍ | 9/200 [00:00<00:02, 85.09it/s]
Step 16: 4%|▍ | 9/200 [00:00<00:02, 85.09it/s]
Step 17: 4%|▍ | 9/200 [00:00<00:02, 85.09it/s]
Step 18: 4%|▍ | 9/200 [00:00<00:02, 85.09it/s]
Step 18: 9%|▉ | 18/200 [00:00<00:02, 86.29it/s]
Step 19: 9%|▉ | 18/200 [00:00<00:02, 86.29it/s]
Step 20: 9%|▉ | 18/200 [00:00<00:02, 86.29it/s]
Step 21: 9%|▉ | 18/200 [00:00<00:02, 86.29it/s]
Step 22: 9%|▉ | 18/200 [00:00<00:02, 86.29it/s]
Step 23: 9%|▉ | 18/200 [00:00<00:02, 86.29it/s]
Step 24: 9%|▉ | 18/200 [00:00<00:02, 86.29it/s]
Step 25: 9%|▉ | 18/200 [00:00<00:02, 86.29it/s]
Step 26: 9%|▉ | 18/200 [00:00<00:02, 86.29it/s]
Step 27: 9%|▉ | 18/200 [00:00<00:02, 86.29it/s]
Step 27: 14%|█▎ | 27/200 [00:00<00:02, 86.37it/s]
Step 28: 14%|█▎ | 27/200 [00:00<00:02, 86.37it/s]
Step 29: 14%|█▎ | 27/200 [00:00<00:02, 86.37it/s]
Step 30: 14%|█▎ | 27/200 [00:00<00:02, 86.37it/s]
Step 31: 14%|█▎ | 27/200 [00:00<00:02, 86.37it/s]
Step 32: 14%|█▎ | 27/200 [00:00<00:02, 86.37it/s]
Step 33: 14%|█▎ | 27/200 [00:00<00:02, 86.37it/s]
Step 34: 14%|█▎ | 27/200 [00:00<00:02, 86.37it/s]
Step 35: 14%|█▎ | 27/200 [00:00<00:02, 86.37it/s]
Step 36: 14%|█▎ | 27/200 [00:00<00:02, 86.37it/s]
Step 36: 18%|█▊ | 36/200 [00:00<00:01, 85.44it/s]
Step 37: 18%|█▊ | 36/200 [00:00<00:01, 85.44it/s]
Step 38: 18%|█▊ | 36/200 [00:00<00:01, 85.44it/s]
Step 39: 18%|█▊ | 36/200 [00:00<00:01, 85.44it/s]
Step 40: 18%|█▊ | 36/200 [00:00<00:01, 85.44it/s]
Step 41: 18%|█▊ | 36/200 [00:00<00:01, 85.44it/s]
Step 42: 18%|█▊ | 36/200 [00:00<00:01, 85.44it/s]
Step 43: 18%|█▊ | 36/200 [00:00<00:01, 85.44it/s]
Step 44: 18%|█▊ | 36/200 [00:00<00:01, 85.44it/s]
Step 45: 18%|█▊ | 36/200 [00:00<00:01, 85.44it/s]
Step 45: 22%|██▎ | 45/200 [00:00<00:01, 83.80it/s]
Step 46: 22%|██▎ | 45/200 [00:00<00:01, 83.80it/s]
Step 47: 22%|██▎ | 45/200 [00:00<00:01, 83.80it/s]
Step 48: 22%|██▎ | 45/200 [00:00<00:01, 83.80it/s]
Step 49: 22%|██▎ | 45/200 [00:00<00:01, 83.80it/s]
Step 50: 22%|██▎ | 45/200 [00:00<00:01, 83.80it/s]
Step 51: 22%|██▎ | 45/200 [00:00<00:01, 83.80it/s]
Step 52: 22%|██▎ | 45/200 [00:00<00:01, 83.80it/s]
Step 53: 22%|██▎ | 45/200 [00:00<00:01, 83.80it/s]
Step 54: 22%|██▎ | 45/200 [00:00<00:01, 83.80it/s]
Step 54: 27%|██▋ | 54/200 [00:00<00:01, 83.18it/s]
Step 55: 27%|██▋ | 54/200 [00:00<00:01, 83.18it/s]
Step 56: 27%|██▋ | 54/200 [00:00<00:01, 83.18it/s]
Step 57: 27%|██▋ | 54/200 [00:00<00:01, 83.18it/s]
Step 58: 27%|██▋ | 54/200 [00:00<00:01, 83.18it/s]
Step 59: 27%|██▋ | 54/200 [00:00<00:01, 83.18it/s]
Step 60: 27%|██▋ | 54/200 [00:00<00:01, 83.18it/s]
Step 61: 27%|██▋ | 54/200 [00:00<00:01, 83.18it/s]
Step 62: 27%|██▋ | 54/200 [00:00<00:01, 83.18it/s]
Step 63: 27%|██▋ | 54/200 [00:00<00:01, 83.18it/s]
Step 63: 32%|███▏ | 63/200 [00:00<00:01, 83.98it/s]
Step 64: 32%|███▏ | 63/200 [00:00<00:01, 83.98it/s]
Step 65: 32%|███▏ | 63/200 [00:00<00:01, 83.98it/s]
Step 66: 32%|███▏ | 63/200 [00:00<00:01, 83.98it/s]
Step 67: 32%|███▏ | 63/200 [00:00<00:01, 83.98it/s]
Step 68: 32%|███▏ | 63/200 [00:00<00:01, 83.98it/s]
Step 69: 32%|███▏ | 63/200 [00:00<00:01, 83.98it/s]
Step 70: 32%|███▏ | 63/200 [00:00<00:01, 83.98it/s]
Step 71: 32%|███▏ | 63/200 [00:00<00:01, 83.98it/s]
Step 72: 32%|███▏ | 63/200 [00:00<00:01, 83.98it/s]
Step 72: 36%|███▌ | 72/200 [00:00<00:01, 84.44it/s]
Step 73: 36%|███▌ | 72/200 [00:00<00:01, 84.44it/s]
Step 74: 36%|███▌ | 72/200 [00:00<00:01, 84.44it/s]
Step 75: 36%|███▌ | 72/200 [00:00<00:01, 84.44it/s]
Step 76: 36%|███▌ | 72/200 [00:00<00:01, 84.44it/s]
Step 77: 36%|███▌ | 72/200 [00:00<00:01, 84.44it/s]
Step 78: 36%|███▌ | 72/200 [00:00<00:01, 84.44it/s]
Step 79: 36%|███▌ | 72/200 [00:00<00:01, 84.44it/s]
Step 80: 36%|███▌ | 72/200 [00:00<00:01, 84.44it/s]
Step 81: 36%|███▌ | 72/200 [00:00<00:01, 84.44it/s]
Step 81: 40%|████ | 81/200 [00:00<00:01, 84.88it/s]
Step 82: 40%|████ | 81/200 [00:00<00:01, 84.88it/s]
Step 83: 40%|████ | 81/200 [00:00<00:01, 84.88it/s]
Step 84: 40%|████ | 81/200 [00:00<00:01, 84.88it/s]
Step 85: 40%|████ | 81/200 [00:01<00:01, 84.88it/s]
Step 86: 40%|████ | 81/200 [00:01<00:01, 84.88it/s]
Step 87: 40%|████ | 81/200 [00:01<00:01, 84.88it/s]
Step 88: 40%|████ | 81/200 [00:01<00:01, 84.88it/s]
Step 89: 40%|████ | 81/200 [00:01<00:01, 84.88it/s]
Step 90: 40%|████ | 81/200 [00:01<00:01, 84.88it/s]
Step 90: 45%|████▌ | 90/200 [00:01<00:01, 84.48it/s]
Step 91: 45%|████▌ | 90/200 [00:01<00:01, 84.48it/s]
Step 92: 45%|████▌ | 90/200 [00:01<00:01, 84.48it/s]
Step 93: 45%|████▌ | 90/200 [00:01<00:01, 84.48it/s]
Step 94: 45%|████▌ | 90/200 [00:01<00:01, 84.48it/s]
Step 95: 45%|████▌ | 90/200 [00:01<00:01, 84.48it/s]
Step 96: 45%|████▌ | 90/200 [00:01<00:01, 84.48it/s]
Step 97: 45%|████▌ | 90/200 [00:01<00:01, 84.48it/s]
Step 98: 45%|████▌ | 90/200 [00:01<00:01, 84.48it/s]
Step 99: 45%|████▌ | 90/200 [00:01<00:01, 84.48it/s]
Step 99: 50%|████▉ | 99/200 [00:01<00:01, 84.01it/s]
Step 100: 50%|████▉ | 99/200 [00:01<00:01, 84.01it/s]
Step 101: 50%|████▉ | 99/200 [00:01<00:01, 84.01it/s]
Step 102: 50%|████▉ | 99/200 [00:01<00:01, 84.01it/s]
Step 103: 50%|████▉ | 99/200 [00:01<00:01, 84.01it/s]
Step 104: 50%|████▉ | 99/200 [00:01<00:01, 84.01it/s]
Step 105: 50%|████▉ | 99/200 [00:01<00:01, 84.01it/s]
Step 106: 50%|████▉ | 99/200 [00:01<00:01, 84.01it/s]
Step 107: 50%|████▉ | 99/200 [00:01<00:01, 84.01it/s]
Step 108: 50%|████▉ | 99/200 [00:01<00:01, 84.01it/s]
Step 108: 54%|█████▍ | 108/200 [00:01<00:01, 83.91it/s]
Step 109: 54%|█████▍ | 108/200 [00:01<00:01, 83.91it/s]
Step 110: 54%|█████▍ | 108/200 [00:01<00:01, 83.91it/s]
Step 111: 54%|█████▍ | 108/200 [00:01<00:01, 83.91it/s]
Step 112: 54%|█████▍ | 108/200 [00:01<00:01, 83.91it/s]
Step 113: 54%|█████▍ | 108/200 [00:01<00:01, 83.91it/s]
Step 114: 54%|█████▍ | 108/200 [00:01<00:01, 83.91it/s]
Step 115: 54%|█████▍ | 108/200 [00:01<00:01, 83.91it/s]
Step 116: 54%|█████▍ | 108/200 [00:01<00:01, 83.91it/s]
Step 117: 54%|█████▍ | 108/200 [00:01<00:01, 83.91it/s]
Step 117: 58%|█████▊ | 117/200 [00:01<00:00, 84.23it/s]
Step 118: 58%|█████▊ | 117/200 [00:01<00:00, 84.23it/s]
Step 119: 58%|█████▊ | 117/200 [00:01<00:00, 84.23it/s]
Step 120: 58%|█████▊ | 117/200 [00:01<00:00, 84.23it/s]
Step 121: 58%|█████▊ | 117/200 [00:01<00:00, 84.23it/s]
Step 122: 58%|█████▊ | 117/200 [00:01<00:00, 84.23it/s]
Step 123: 58%|█████▊ | 117/200 [00:01<00:00, 84.23it/s]
Step 124: 58%|█████▊ | 117/200 [00:01<00:00, 84.23it/s]
Step 125: 58%|█████▊ | 117/200 [00:01<00:00, 84.23it/s]
Step 126: 58%|█████▊ | 117/200 [00:01<00:00, 84.23it/s]
Step 126: 63%|██████▎ | 126/200 [00:01<00:00, 84.02it/s]
Step 127: 63%|██████▎ | 126/200 [00:01<00:00, 84.02it/s]
Step 128: 63%|██████▎ | 126/200 [00:01<00:00, 84.02it/s]
Step 129: 63%|██████▎ | 126/200 [00:01<00:00, 84.02it/s]
Step 130: 63%|██████▎ | 126/200 [00:01<00:00, 84.02it/s]
Step 131: 63%|██████▎ | 126/200 [00:01<00:00, 84.02it/s]
Step 132: 63%|██████▎ | 126/200 [00:01<00:00, 84.02it/s]
Step 133: 63%|██████▎ | 126/200 [00:01<00:00, 84.02it/s]
Step 134: 63%|██████▎ | 126/200 [00:01<00:00, 84.02it/s]
Step 135: 63%|██████▎ | 126/200 [00:01<00:00, 84.02it/s]
Step 135: 68%|██████▊ | 135/200 [00:01<00:00, 83.92it/s]
Step 136: 68%|██████▊ | 135/200 [00:01<00:00, 83.92it/s]
Step 137: 68%|██████▊ | 135/200 [00:01<00:00, 83.92it/s]
Step 138: 68%|██████▊ | 135/200 [00:01<00:00, 83.92it/s]
Step 139: 68%|██████▊ | 135/200 [00:01<00:00, 83.92it/s]
Step 140: 68%|██████▊ | 135/200 [00:01<00:00, 83.92it/s]
Step 141: 68%|██████▊ | 135/200 [00:01<00:00, 83.92it/s]
Step 142: 68%|██████▊ | 135/200 [00:01<00:00, 83.92it/s]
Step 143: 68%|██████▊ | 135/200 [00:01<00:00, 83.92it/s]
Step 144: 68%|██████▊ | 135/200 [00:01<00:00, 83.92it/s]
Step 144: 72%|███████▏ | 144/200 [00:01<00:00, 81.24it/s]
Step 145: 72%|███████▏ | 144/200 [00:01<00:00, 81.24it/s]
Step 146: 72%|███████▏ | 144/200 [00:01<00:00, 81.24it/s]
Step 147: 72%|███████▏ | 144/200 [00:01<00:00, 81.24it/s]
Step 148: 72%|███████▏ | 144/200 [00:01<00:00, 81.24it/s]
Step 149: 72%|███████▏ | 144/200 [00:01<00:00, 81.24it/s]
Step 150: 72%|███████▏ | 144/200 [00:01<00:00, 81.24it/s]
Step 151: 72%|███████▏ | 144/200 [00:01<00:00, 81.24it/s]
Step 152: 72%|███████▏ | 144/200 [00:01<00:00, 81.24it/s]
Step 153: 72%|███████▏ | 144/200 [00:01<00:00, 81.24it/s]
Step 153: 76%|███████▋ | 153/200 [00:01<00:00, 82.07it/s]
Step 154: 76%|███████▋ | 153/200 [00:01<00:00, 82.07it/s]
Step 155: 76%|███████▋ | 153/200 [00:01<00:00, 82.07it/s]
Step 156: 76%|███████▋ | 153/200 [00:01<00:00, 82.07it/s]
Step 157: 76%|███████▋ | 153/200 [00:01<00:00, 82.07it/s]
Step 158: 76%|███████▋ | 153/200 [00:01<00:00, 82.07it/s]
Step 159: 76%|███████▋ | 153/200 [00:01<00:00, 82.07it/s]
Step 160: 76%|███████▋ | 153/200 [00:01<00:00, 82.07it/s]
Step 161: 76%|███████▋ | 153/200 [00:01<00:00, 82.07it/s]
Step 162: 76%|███████▋ | 153/200 [00:01<00:00, 82.07it/s]
Step 162: 81%|████████ | 162/200 [00:01<00:00, 83.20it/s]
Step 163: 81%|████████ | 162/200 [00:01<00:00, 83.20it/s]
Step 164: 81%|████████ | 162/200 [00:01<00:00, 83.20it/s]
Step 165: 81%|████████ | 162/200 [00:01<00:00, 83.20it/s]
Step 166: 81%|████████ | 162/200 [00:01<00:00, 83.20it/s]
Step 167: 81%|████████ | 162/200 [00:01<00:00, 83.20it/s]
Step 168: 81%|████████ | 162/200 [00:02<00:00, 83.20it/s]
Step 169: 81%|████████ | 162/200 [00:02<00:00, 83.20it/s]
Step 170: 81%|████████ | 162/200 [00:02<00:00, 83.20it/s]
Step 171: 81%|████████ | 162/200 [00:02<00:00, 83.20it/s]
Step 171: 86%|████████▌ | 171/200 [00:02<00:00, 83.78it/s]
Step 172: 86%|████████▌ | 171/200 [00:02<00:00, 83.78it/s]
Step 173: 86%|████████▌ | 171/200 [00:02<00:00, 83.78it/s]
Step 174: 86%|████████▌ | 171/200 [00:02<00:00, 83.78it/s]
Step 175: 86%|████████▌ | 171/200 [00:02<00:00, 83.78it/s]
Step 176: 86%|████████▌ | 171/200 [00:02<00:00, 83.78it/s]
Step 177: 86%|████████▌ | 171/200 [00:02<00:00, 83.78it/s]
Step 178: 86%|████████▌ | 171/200 [00:02<00:00, 83.78it/s]
Step 179: 86%|████████▌ | 171/200 [00:02<00:00, 83.78it/s]
Step 180: 86%|████████▌ | 171/200 [00:02<00:00, 83.78it/s]
Step 180: 90%|█████████ | 180/200 [00:02<00:00, 84.52it/s]
Step 181: 90%|█████████ | 180/200 [00:02<00:00, 84.52it/s]
Step 182: 90%|█████████ | 180/200 [00:02<00:00, 84.52it/s]
Step 183: 90%|█████████ | 180/200 [00:02<00:00, 84.52it/s]
Step 184: 90%|█████████ | 180/200 [00:02<00:00, 84.52it/s]
Step 185: 90%|█████████ | 180/200 [00:02<00:00, 84.52it/s]
Step 186: 90%|█████████ | 180/200 [00:02<00:00, 84.52it/s]
Step 187: 90%|█████████ | 180/200 [00:02<00:00, 84.52it/s]
Step 188: 90%|█████████ | 180/200 [00:02<00:00, 84.52it/s]
Step 189: 90%|█████████ | 180/200 [00:02<00:00, 84.52it/s]
Step 189: 94%|█████████▍| 189/200 [00:02<00:00, 85.54it/s]
Step 190: 94%|█████████▍| 189/200 [00:02<00:00, 85.54it/s]
Step 191: 94%|█████████▍| 189/200 [00:02<00:00, 85.54it/s]
Step 192: 94%|█████████▍| 189/200 [00:02<00:00, 85.54it/s]
Step 193: 94%|█████████▍| 189/200 [00:02<00:00, 85.54it/s]
Step 194: 94%|█████████▍| 189/200 [00:02<00:00, 85.54it/s]
Step 195: 94%|█████████▍| 189/200 [00:02<00:00, 85.54it/s]
Step 196: 94%|█████████▍| 189/200 [00:02<00:00, 85.54it/s]
Step 197: 94%|█████████▍| 189/200 [00:02<00:00, 85.54it/s]
Step 198: 94%|█████████▍| 189/200 [00:02<00:00, 85.54it/s]
Step 198: 99%|█████████▉| 198/200 [00:02<00:00, 86.16it/s]
Step 199: 99%|█████████▉| 198/200 [00:02<00:00, 86.16it/s]
Step 200: 99%|█████████▉| 198/200 [00:02<00:00, 86.16it/s]
Step 200: 100%|██████████| 200/200 [00:02<00:00, 84.39it/s]
PSNRs:
Filtered Backprojection: 24.22
EPLL: 32.33
PatchNR: 32.89
Total running time of the script: (0 minutes 15.675 seconds)